Getting started in React with Redux
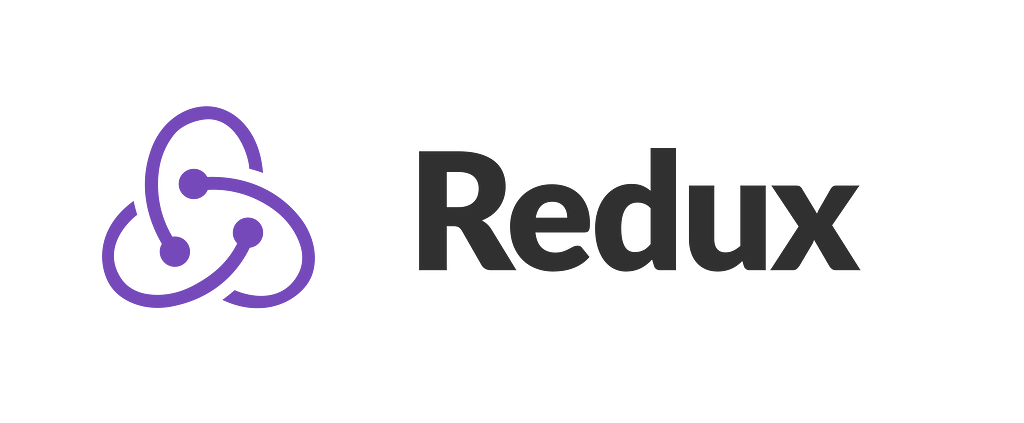
React is lightweight compared to similar front-end technologies (it’s just the V in your typical MVC), so it requires a lot of supporting libraries. One of the most commonly used is the state management system Redux.
Redux is deceptively small, even on the github repository it says: “It is tiny (2kB, including dependencies)”. It has a simple premise, but there’s plenty to learn in order to use it properly, especially in large applications. Here are some of the key points I learned, using it for the first time.
Redux won’t take care of your model structure for you
Redux deals with model state, but it doesn’t provide commonly needed functionality like: preparing and parsing data from the backend, data validation, and change detection. You’ll either need to bring in supporting libraries, or if your project is small enough you can take care of it on a case by case basis.
Not all of your state should be in Redux
Redux emphasises there being a single source of truth for your data. This is hard when you’re dealing with the DOM which already has a representation of it. Sometimes leaving this data in the DOM is sufficient (think of a toggle switch with enabled/disabled states) as bringing that property into Redux may unnecessarily complicate things.
You can try to group related properties
When your state grows it means you’ll likely want to group properties in order to identify them faster. If you’ve decided you want to store UI state (related to the point above), I’ve found grouping properties under ‘ui’ and ‘data’ to be nice starting point.
You should use container components
Container components serve as the connection between your React components and Redux. They abstract the state management of Redux so your components don’t have to know how redux is managing your state. If your app is at least moderately sized, I would consider saving yourself the headache and using container components from the start.
Structuring your reducers well is important
When starting out it’s fine to write all of your reducer logic in one file. But in the future you’ll probably want to separate distinct areas of your application into different files using the function ‘combineReducers’ supplied by redux.
There are many ways of structuring your Redux files, a common paradigm is called Ducks. Ducks is a good starting point, but finding a good solution to your project size and needs is a complex problem, and can greatly affect your productivity. I’d recommend reading up on some of the advice in the official documentation.
Selectors can help with structuring your reducers
Selectors work by specifying the local area of the state you want to work on. This helps by abstracting away unneeded parts of the state, and gives you more flexibility. You need to bring in a library to make use of selectors, the most popular is called Reselect. Here’s a selector being used in action
You should use Redux DevTools for debugging
Because the state managed by redux isn’t directly tied to the UI where it’s visible, it’s great to have a tool that lets you inspect both the entire structure as well as diffs between actions. Installation instructions for different browsers are found here.
You might want to use Immutable.js
It’s important in Redux for previous states to be immutable due to a number of factors. Using a library like Immutable.js to ensure previous states aren’t modified is great, but I find the API cumbersome to work with. Although I haven’t used it yet, seamless-immutable seems to solve this problem so that you can use immutable data structures, while still preserving the old syntax.
Conclusion
Redux surprised me with how many supporting libraries and contextual knowledge was required to use it efficiently. Especially as it’s so small itself.
I find with redux there’s not often a single way to do things, which is reflected in my tentative answers above. Hopefully these points give you a solid foundation for starting your next project in React with Redux.