Debugging Javascript Callbacks with the Chrome Debugger
I recently worked on a bug within isolated and untested code written with a functional reactive programming language Bacon.js. The following are observations and experiences learned.
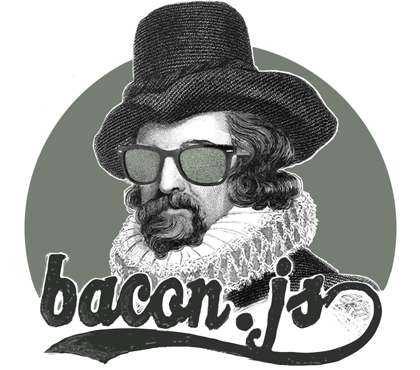
As with most bugs it’s simpler to isolate single input elements in order to divide and conquer. Ensure to solve one element or event at a time.
For me this element was hidden which didn’t affect functionality but made it harder to debug. I found this was easiest made visible by running $(“.myClass”).attr(“type=’text’”) in the console after each page refresh.
Adding logging to event handlers such as $($0).change(function( console.log(“changed”); ); can help in debugging user interaction, but unfortunately doesn’t trigger when the input is programatically set which is the case with callbacks.
There can be issues when debugging blur events if your breakpoint is too generic and triggers on both focus and blur. When the breakpoint triggers on focus, focus will then be given to the console which will prevent you from triggering the blur event.
You can solve this by being more specific with your breakpoints or creating conditional breakpoints that contain the event explicitly. event.type === focus works well.
If you have access to the event, but not it’s origin — Event.target is a useful property that will tell you the element origin. This will give you clues on why the event was fired.
There are multiple ways that event handlers can be specified on an element. Some can be subtly added by a css class which is picked up in an external library, others can be more obviously defined within your codebase itself.
Events in Javascript are complex yet prevalent, so it’s important to understand their complexities. I learnt a lot from debugging this particular bug.